Create Bleutooth Low Energy Application with C# and BleuIO
January 5, 2022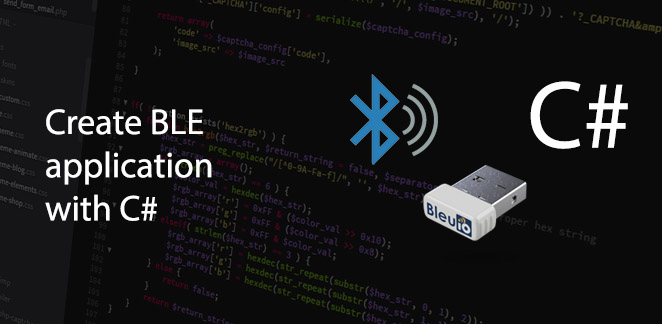
Bluetooth Low Energy (BLE) is a low power wireless technology used for connecting devices with each other. It is a popular communication method especially in the era of Internet of Things. Several devices around the house have a build-in buetooth transceiver and most of them provide really useful capabilitites to automate jobs. For that reason it is really interesting to be able to create a main-device (like a PC) which will have an application that connects to the devices around the house and manage them.
In this example we are going to create a simple C# console application to communicate with BleuIO using SerialPort. This script can be used to create Bluetooth Low Energy application using C# with BleuIO.
Let’s start
As a first step lets create a new project in visual studio and select C# console application from the list.

Choose a suitable name for your project and paste the following code to Program.cs file.
The source code is available at github.
using System;
using System.IO.Ports;
using System.Text;
namespace SerialPortExample
{
class SerialPortProgram
{
static SerialPort _port;
static void Main(string[] args)
{
string portName;
portName = SetPortName();
_port = new SerialPort(portName,57600, Parity.None, 8, StopBits.One);
// Instatiate this class
SerialPortProgram serialPortProgram = new SerialPortProgram();
}
private SerialPortProgram()
{
_port.DataReceived += new
SerialDataReceivedEventHandler(port_DataReceived);
OpenMyPort();
Console.WriteLine("Type q to exit.");
bool continueLoop = true;
string inputCmd;
while (continueLoop)
{
inputCmd = Console.ReadLine();
if (inputCmd == "q")
{
continueLoop = false;
break;
}
else
{
byte[] bytes = Encoding.UTF8.GetBytes(inputCmd);
var inputByte = new byte[] { 13 };
bytes = bytes.Concat(inputByte).ToArray();
_port.Write(bytes, 0, bytes.Length);
}
}
}
private void port_DataReceived(object sender,
SerialDataReceivedEventArgs e)
{
// Show all the incoming data in the port's buffer
Console.WriteLine(_port.ReadExisting());
}
//Open selected COM port
private static void OpenMyPort()
{
try
{
_port.Open();
}
catch (Exception ex)
{
Console.WriteLine("Error opening my port: {0}", ex.Message);
Environment.Exit(0);
}
}
// Display Port values and prompt user to enter a port.
public static string SetPortName()
{
string portName;
Console.WriteLine("Available Ports:");
foreach (string s in SerialPort.GetPortNames())
{
Console.WriteLine(" {0}", s);
}
Console.Write("Enter COM port value (ex: COM18): ");
portName = Console.ReadLine();
return portName;
}
}
}
For this script a BleuIO dongle connected to the computer.
When we run the application, it suggests all the available ports on the computer and asks to write the com port number where the BleuIO is connected.
Once we write the correct port number, it starts communicating with BleuIO via SerialPort.
The response of AT commands from the BleuIO will be shown on the screen.
Find the list of available AT commands at BleuIO getting started guide.
The application will terminate with control+c or type ‘q’.
Facebook Twitter LinkedIn